Jquery Run Function Check Variable Then Run Again if True

There will exist times where y'all will desire to write commands that handle unlike decisions in your code.
For case, if you lot are coding a bot, y'all can have it answer with different messages based on a set of commands it receives.
In this article, I will explain what an if...else
argument is and provide lawmaking examples. We will also await at the conditional (ternary) operator which yous can use equally a autograph for the if...else
statement.
What is an if...else statement in JavaScript?
The if...else
is a type of conditional statement that volition execute a block of code when the condition in the if
statement is truthy
. If the status is falsy
, so the else
block will be executed.
Truthy
and falsy
values are converted to true
or false
inif
statements.
if (condition is true) { // lawmaking is executed } else { // code is executed }
Any value that is non defined equally falsy
would be considered truthy
in JavaScript.
Here is a list offalsy
values:
- false
- 0 (zero)
- -0 (negative zero)
- 0n (BigInt zero)
-
""
,''
,``
(empty string) - null
- undefined
- NaN (not a number)
Examples of if...else statements in JavaScript
In this instance, the condition for the if
statement is true
so the message printed to the console would be "Nick is an adult."
const historic period = xviii; if (age >= 18) { console.log("Nick is an adult."); } else { console.log("Nick is a child."); }
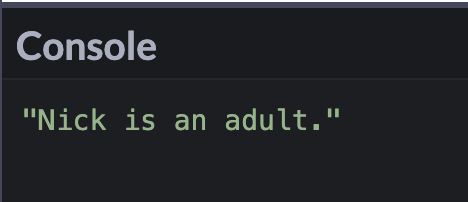
But if I modify the age
variable to be less than 18, then the condition would be imitation
and the code would execute the else
block instead.
const age = 12; if (historic period >= 18) { console.log("Nick is an developed."); } else { console.log("Nick is a child."); }

Examples of multiple weather (if...else if...else statements) in JavaScript
There will exist times where you lot want to test multiple weather condition. That is where the else if
block comes in.
if (status 1 is truthful) { // lawmaking is executed } else if (condition 2 is truthful) { // code is executed } else { // code is executed }
When the if
argument is false
, the computer will move onto the else if
argument. If that is besides false
, and so it will motion onto the else
block.
In this case, the else if
block would be executed considering Alice is between the ages of 18 and 21.
const age = eighteen; if (age < eighteen) { console.log("Alice is nether 18 years one-time."); } else if (age >= xviii && age <= 21) { console.log("Alice is between the ages of 18 and 21."); } else { panel.log("Alice is over 21 years old."); }

When to use switch statements over if...else statements?
There are times in JavaScript where you might consider using a switch
argument instead of an if else
statement.
switch
statements tin have a cleaner syntax over complicated if else
statements.
Take a look at the example below – instead of using this long if else
statement, you might choose to go with an easier to read switch
statement.
const pet = "domestic dog"; if (pet === "lizard") { console.log("I own a cadger"); } else if (pet === "dog") { console.log("I own a dog"); } else if (pet === "cat") { panel.log("I own a cat"); } else if (pet === "snake") { console.log("I own a snake"); } else if (pet === "parrot") { console.log("I own a parrot"); } else { panel.log("I don't own a pet"); }
const pet = "dog"; switch (pet) { case "lizard": console.log("I own a lizard"); suspension; case "dog": panel.log("I own a dog"); break; case "cat": console.log("I own a cat"); break; case "serpent": console.log("I own a ophidian"); break; case "parrot": console.log("I own a parrot"); break; default: console.log("I don't ain a pet"); break; }
switch
statements will non be advisable to use in all situations. Just if you experience like the if else
statements are long and complicated, then a switch
statement could be an alternative option.
The logical AND (&&) operator and if...else statements in JavaScript
In the logical AND (&&
) operator, if both conditions are true
, then the if
cake will be executed. If one or both of the conditions are simulated
, and so the else
block will exist executed.
In this case, since historic period is greater than 16 and the ownsCar
variable is truthful
, the if
cake volition run. The message printed to the console will be "Jerry is old plenty to drive and has his ain car."
const historic period = 17; const ownsCar = true; if (age >= 16 && ownsCar) { console.log("Jerry is old enough to drive and has his own auto."); } else { panel.log("Jerry does non drive."); }

If I modify the age
variable to exist less than sixteen, then both conditions are no longer true
and the else
block would be executed instead.
const age = 13; const ownsCar = true; if (age >= 16 && ownsCar) { console.log("Jerry is old enough to drive and has his own motorcar."); } else { console.log("Jerry does not drive."); }
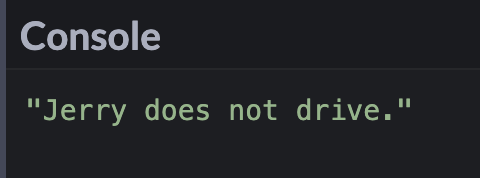
The logical OR (||) operator and if...else statements in JavaScript
In the logical OR (||
) operator, if 1 or both of the weather condition are truthful
, then the code inside the if
statement will execute.
In this example, even though the isSale
variable is set to faux
, the code inside the if
block will still execute considering the boyfriendIsPaying
variable is fix to true
.
const boyfriendIsPaying = true; const isSale = simulated; if (boyfriendIsPaying || isSale) { console.log("Jesse will become shopping."); } else { console.log("Jesse will non get shopping."); }

If I were to modify the value of the boyfriendIsPaying
variable to false
, and so the else
cake would execute because both conditions are false
.
const boyfriendIsPaying = false; const isSale = faux; if (boyfriendIsPaying || isSale) { console.log("Jesse will go shopping."); } else { console.log("Jesse will not get shopping."); }

The logical NOT (!) operator and if...else statements in JavaScript
The logical NOT (!
) operator will take something that is true
and make it simulated
. Information technology will besides take something that is false
and make it true
.
We can alter the example from earlier to employ the !
operator to make the boyfriendIsPaying
variablefalse
. Since both weather condition are simulated
, the else
block would be executed.
const boyfriendIsPaying = true; const isSale = faux; if (!boyfriendIsPaying || isSale) { panel.log("Jesse volition go shopping."); } else { console.log("Jesse volition not go shopping."); }
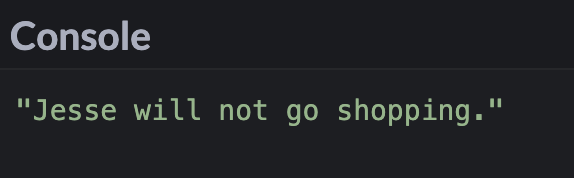
Conditional (ternary) operator in JavaScript
If you lot have a short if else
statement, then you might choose to go with the ternary operator. The discussion ternary ways something composed of iii parts.
This is the bones syntax for a ternary operator:
condition ? if condition is true : if condition is faux
The condition goes before the ?
mark and if it is true
, then the lawmaking betwixt the ?
marking and :
would execute. If the condition is false
, then the code after the:
would execute.
In this example, since age is greater than 18, then the bulletin to the console would be "Can vote".
const age = 32; const citizen = age >= xviii ? "Can vote" : "Cannot vote"; console.log(citizen);

This is what the code would look like using an if else
statement:
const historic period = 32; let citizen; if (age >= 18) { citizen = "Can vote"; } else { denizen = "Cannot vote"; } console.log(citizen);
Decision
if else
statements will execute a block of code when the condition in the if
statement is truthy
. If the status is falsy
, then the else
block will be executed.
There will be times where yous desire to exam multiple conditions and you tin can use an if...else if...else
argument.
If you experience like the if else
argument is long and complicated, then a switch
statement could exist an alternative option.
Using logical operators to test multiple weather can supercede nested if else
statements.
The ternary operator can be used to write shorter code for a elementary if else
statement.
Learn to code for complimentary. freeCodeCamp'due south open source curriculum has helped more than 40,000 people get jobs as developers. Get started
davidsonforeplarks.blogspot.com
Source: https://www.freecodecamp.org/news/javascript-if-else-and-if-then-js-conditional-statements/
0 Response to "Jquery Run Function Check Variable Then Run Again if True"
Post a Comment